1. Overview
In this tutorial, We'll discuss how to use Predicate with Optional class. The Java 8 Optional class has a method filter() which takes Predicate as an argument.Optional is a class and it is in java.util package. Optional is declared as final in its source code. Because no other classes can be inherited and to stop overriding the behavior.
API Note: If a value is present, and the value matches the given predicate, returns an Optional describing the value, otherwise returns an empty Optional.
First, let us take a look at syntax, it's explanation, and example programs.
Java 8 Optional filter()
2. filter(Predicate predicate) Syntax
Below is the syntax for filter() method.public Optional<T> filter(Predicate<? super T> predicate)
filter() method accepts Predicate as an argument and returns Optional value.
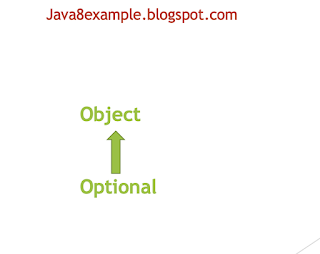
Predicate Example:
Predicate takes one argument and returns boolean. Checks for a condition and returns true if condition satisfies else false.
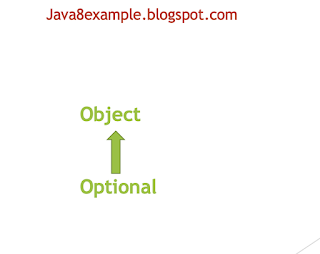
Predicate Example:
Predicate takes one argument and returns boolean. Checks for a condition and returns true if condition satisfies else false.
Predicate<Employee> empSal = e -> e.getSal() > 10000;
This checks for the condition employee salary > 10000.
Below is the example program on filter() method.
In the example, first created an Optional instance by calling of() method with the string "Hello Mate, Welcome to java8example blog".
Next, created a predicate welcomePredicate to validate if the string contains the "Welcome" word in it. After this, using filter(welcomePredicate) method and passed welcomePredicate as an argument. When the filter() method is executed, welcomePredicate is evaluated. If welcomePredicate is true then it returns the current string.
In the next example, Created another Predicate haiPredicate to validate if the string contains "Hai". Again called the Optional filter(haiPredicate) and evaluated haiPredicate. But no "Hai" text found. Hence, the condition in haiPredicate is failed. So it returned empty optional instance. because of this, the output is printed as "Optional.empty".
3. Java 8 filter() Example for String
Below is the example program on filter() method.
In the example, first created an Optional instance by calling of() method with the string "Hello Mate, Welcome to java8example blog".
Next, created a predicate welcomePredicate to validate if the string contains the "Welcome" word in it. After this, using filter(welcomePredicate) method and passed welcomePredicate as an argument. When the filter() method is executed, welcomePredicate is evaluated. If welcomePredicate is true then it returns the current string.
In the next example, Created another Predicate haiPredicate to validate if the string contains "Hai". Again called the Optional filter(haiPredicate) and evaluated haiPredicate. But no "Hai" text found. Hence, the condition in haiPredicate is failed. So it returned empty optional instance. because of this, the output is printed as "Optional.empty".
package com.java.w3schools.blog.java8.optional; import java.util.Optional; import java.util.function.Predicate; /** * java 8 Optional filter() method example * * @author Venkatesh * */ public class OptionalFilterExample { public static void main(String[] args) { Optional<String> helloOptonal = Optional.of("Hello Mate, Welcome to java8example blog"); // Predicate match case Predicate<String> welcomePredicate = s -> s.contains("Welcome"); Optional<String> welcomeOptional = helloOptonal.filter(welcomePredicate); System.out.println("welcomeOptional : " + welcomeOptional); // Predicate non-match case Predicate<String> haiPredicate = s -> s.contains("Hai"); Optional<String> haiOptional = helloOptonal.filter(haiPredicate); System.out.println("haiOptional : " + haiOptional); } }
Output:
welcomeOptional : Optional[Hello Mate, Welcome to java8example blog] haiOptional : Optional.empty
4. filter() Example but Passing List to Optional
In the above example, First created an Optional instance with String. In this example, Now we will create an Optional with List. We'll see how filter method behaves.
List<String> countries = Arrays.asList("USA", "UK", "AUS"); Optional<List<String>> countriesOptional = Optional.of(countries); Predicate<List<String>> listPredicate = list -> list.stream().filter(name -> name.startsWith("U")).count() > 0; Optional listOpional = countriesOptional.filter(listPredicate); System.out.println("List Optional filter() : "+listOpional);
Output:
List Optional filter() : Optional[[USA, UK, AUS]]
5. Internal Implementation
Below is the internal implementation code from java 12 API.
public Optional<T> filter(Predicate<? super T> predicate) { Objects.requireNonNull(predicate); if (!isPresent()) { return this; } else { return predicate.test(value) ? this : empty(); } }
Internally, it calls predicate.test(value) method where test() is a functional method.
In this article, We've discussed Optional.filter() method with examples. filter() method takes Predicate as an argument and returns the Optional object if the Predicate condition is true.
This works the same for String, List, Map, or any object that Optional object is formed.
And also seen on how it works internally and what method is invoked to validate the predicate.
If we pass the predicate is null, it throws NullPointerException.
Full example code on GitHub
API Ref
6. Conclusion
In this article, We've discussed Optional.filter() method with examples. filter() method takes Predicate as an argument and returns the Optional object if the Predicate condition is true.
This works the same for String, List, Map, or any object that Optional object is formed.
And also seen on how it works internally and what method is invoked to validate the predicate.
If we pass the predicate is null, it throws NullPointerException.
Full example code on GitHub
API Ref
No comments:
Post a Comment
Please do not add any spam links in the comments section.